Creating schemas
API reference available here.
This article provides an example of creating a simple schema, with most formatting parameters left at default values.
Request example
The example creates a form with five fields for data:
- Employee ID
- First name
- Last name
- Information if the employee is a contractor
- Information about days off left to use this year
The details are explained further in this article.
curl --location --request \
POST 'https://{SYNERISE_API_BASE_PATH}/boxes-v2/schemas' \
--header 'api-version: 4.2' \
--header 'authorization: Bearer eyJdG...loFAnPY' \
--header 'Content-Type: application/json' \
--data-raw '{
"schemaName": "Employee data",
"tags": [],
"fields": {
"daysOff": {
"type": "NUMBER",
"data": {
"decimalPlaces": 1
},
"design": {
"description": "How many days off the employee has available this year",
"hideLabel": false,
"initialFocus": false,
"label": "Days off left",
"placeholder": "Enter value",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"validation": {
"errorMessage": "Enter a valid number",
"maximumValue": 100000.0,
"minimumValue": 0.0,
"regexPattern": null,
"required": false,
"unique": false
}
},
"lastName": {
"type": "TEXT",
"design": {
"description": "",
"hideLabel": false,
"initialFocus": false,
"label": "Last name",
"placeholder": "",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"validation": {
"errorMessage": "",
"maximumLength": null,
"minimumLength": null,
"regexPattern": null,
"required": true,
"unique": false
}
},
"firstName": {
"type": "TEXT",
"design": {
"description": "",
"hideLabel": false,
"initialFocus": false,
"label": "First name",
"placeholder": "",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"validation": {
"errorMessage": "",
"maximumLength": null,
"minimumLength": null,
"regexPattern": null,
"required": true,
"unique": false
}
},
"employeeId": {
"type": "TEXT",
"design": {
"description": "The ID of the employee",
"hideLabel": false,
"initialFocus": true,
"label": "Employee ID",
"placeholder": "ID",
"tabIndex": 0,
"tooltip": "The ID is included in the email address"
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"validation": {
"errorMessage": "",
"maximumLength": null,
"minimumLength": null,
"regexPattern": null,
"required": true,
"unique": false
}
},
"isContractor": {
"type": "SWITCH",
"data": {
"defaultValue": false
},
"design": {
"description": "Toggle on if the person is a contractor",
"hideLabel": false,
"initialFocus": false,
"label": "Contractor",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
}
}
},
"layout": [
{
"type": "SINGLE",
"fieldName": "employeeId"
},
{
"type": "TABLE",
"cells": [
[
[
{
"fieldName": "firstName",
"type": "SINGLE"
}
],
[
{
"fieldName": "lastName",
"type": "SINGLE"
}
]
],
[
[
{
"fieldName": "isContractor",
"type": "SINGLE"
}
],
[
{
"fieldName": "daysOff",
"type": "SINGLE"
}
]
]
],
"design": {
"display": {
"customCssClass": "",
"isBordered": true,
"isCondensed": false,
"isHover": false,
"isStriped": false
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
}
}
}
]
}'
Response
If the request was successful, the response includes the details of the schema, including its UUID, used as the identifier for the schema in other requests.
{
"modifiedAt": "2020-10-26T17:42:11.086Z",
"modifiedBy": 140,
"relations": {},
"schemaId": "3214aca5-d55e-4b0f-8784-e84eae291cf4",
"schemaName": "Employee data"
"businessProfileId": 100005,
"createdAt": "2020-10-26T17:42:11.086Z",
"createdBy": 140,
"deletedAt": null,
"deletedBy": null,
"tags": [],
"fields": {
"isContractor": {
"data": {
"defaultValue": false
},
"design": {
"description": "Toggle on if the person is a contractor",
"hideLabel": false,
"initialFocus": false,
"label": "Contractor",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"type": "SWITCH"
},
"lastName": {
"design": {
"description": "",
"hideLabel": false,
"initialFocus": false,
"label": "Last name",
"placeholder": "",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"type": "TEXT",
"validation": {
"errorMessage": "",
"maximumLength": null,
"minimumLength": null,
"regexPattern": null,
"required": true,
"unique": false
}
},
"daysOff": {
"data": {
"decimalPlaces": 1
},
"design": {
"description": "How many days off the employee has available this year",
"hideLabel": false,
"initialFocus": false,
"label": "Days off left",
"placeholder": "Enter value",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"type": "NUMBER",
"validation": {
"errorMessage": "Enter a valid number",
"maximumValue": 100000.0,
"minimumValue": 0.0,
"regexPattern": null,
"required": false,
"unique": false
}
},
"firstName": {
"design": {
"description": "",
"hideLabel": false,
"initialFocus": false,
"label": "First name",
"placeholder": "",
"tabIndex": 0,
"tooltip": ""
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"type": "TEXT",
"validation": {
"errorMessage": "",
"maximumLength": null,
"minimumLength": null,
"regexPattern": null,
"required": true,
"unique": false
}
},
"employeeId": {
"design": {
"description": "The ID of the employee",
"hideLabel": false,
"initialFocus": true,
"label": "Employee ID",
"placeholder": "ID",
"tabIndex": 0,
"tooltip": "The ID is included in the email address"
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"type": "TEXT",
"validation": {
"errorMessage": "",
"maximumLength": null,
"minimumLength": null,
"regexPattern": null,
"required": true,
"unique": false
}
}
},
"layout": [
{
"fieldName": "employeeId",
"type": "SINGLE"
},
{
"cells": [
[
[
{
"fieldName": "firstName",
"type": "SINGLE"
}
],
[
{
"fieldName": "lastName",
"type": "SINGLE"
}
]
],
[
[
{
"fieldName": "isContractor",
"type": "SINGLE"
}
],
[
{
"fieldName": "daysOff",
"type": "SINGLE"
}
]
]
],
"design": {
"display": {
"customCssClass": "",
"isBordered": true,
"isCondensed": false,
"isHover": false,
"isStriped": false
},
"layout": {
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
}
},
"type": "TABLE"
}
]
}
Errors
If the request fails, the response provides details on what went wrong. For example, when the JSON data includes a syntax error:
The request content was malformed:
Unexpected character '"' at input index 115 (line 6, position 13), expected '}':
"data": {
^
Result in GUI
The figure presents what the form looks like in the Synerise Portal when a user wants to add a data record using this schema. You can also create your own GUI or use no GUI at all and work using only the API.
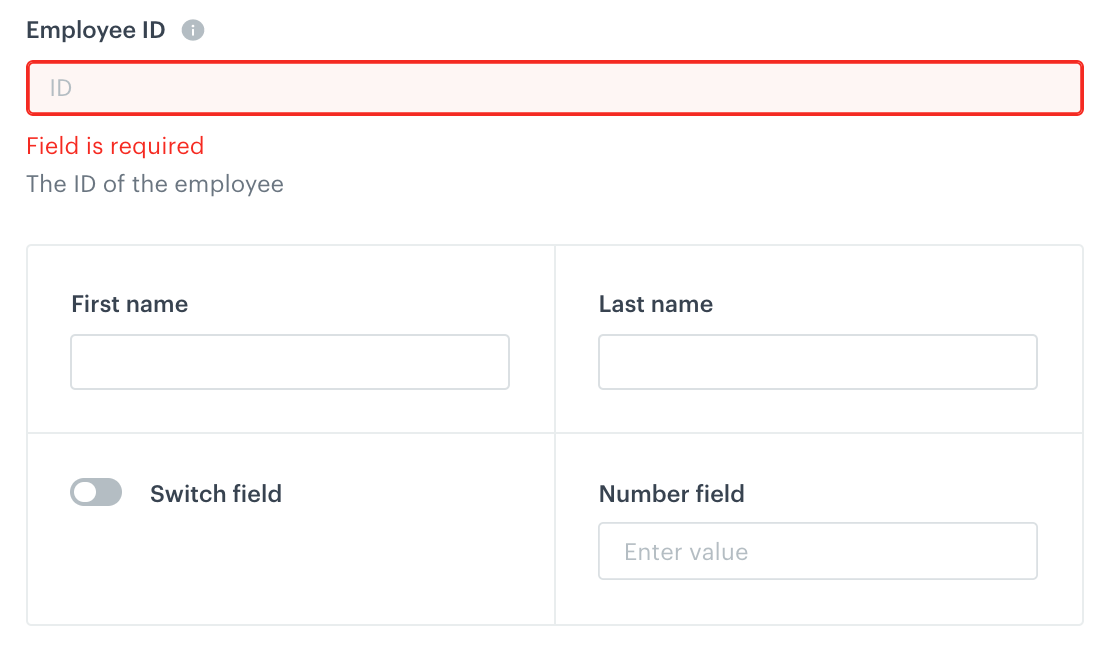
Explanation
Let’s analyze the JSON data object by object.
fields
The fields
object stores the following information about each data field:
- name (unique identifier of a field)
- data type
- data validation
- additional data for the GUI, such as the label of the field
- margins of the field
It does not contain any information about where a field is located in the form. The order of the fields in this object does not affect anything.
The first field defined is the number of days off remaining:
"fields": {
"daysOff": { // unique identifier of the field
"type": "NUMBER", // the field accepts a numeric value
"data": { // additional options, depending on data type, see API reference
"decimalPlaces": 1 // assuming that an employee can take half a day off,
// one decimal place is required
},
"design": { // additional data for the GUI, see API reference for details
"description": "How many days off the employee has available this year",
"hideLabel": false,
"initialFocus": false,
"label": "Days off left",
"placeholder": "Enter value",
"tabIndex": 0,
"tooltip": ""
},
"layout": { // layout within the parent element, default values
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
},
"validation": { // validation depending on data type
"errorMessage": "Enter a valid number",
"maximumValue": 100000.0,
"minimumValue": 0.0,
"regexPattern": null,
"required": false,
"unique": false
}
},
"lastName": { ... }
layout
This array creates the layout of the form, configuring the position of the fields in the form. The position in the array defines the position in the GUI - the layouts are rendered from the top, starting with the first one in the array.
You can use three types of layout elements:
SINGLE
: A single field is the last object in each layout structure. It must a reference to a data entry field from thefields
object.TABLE
: A table may contain other tables, single fields, or columns. There is no limit to how deep layouts can be nested.COLUMNS
: Columns can contain more columns, tables, or single fields. There is no limit to how deep layouts can be nested. Functionally, a column is a single-row table.
SINGLE
layouts).SINGLE
"layout": [
{
"type": "SINGLE" // field type
"fieldName": "employeeId" // unique identifier of the field
},
{
"type": "TABLE"
...
}
]
TABLE
A table object contains information about the fields in the table. The example below is a table with two rows and two columns. Each cell in this example contains one data entry field, but you can create cells with more fields, or cells that contain tables or columns.
The number of columns in all rows must be the same.
{
"type": "TABLE"
"cells": [ // array of rows
[ // first row
[ // first column from the left
{ // data entry field in the cell
"fieldName": "firstName",
"type": "SINGLE"
}
],
[ // second column
{
"fieldName": "lastName",
"type": "SINGLE"
}
]
],
[ // second row
[
{
...
}
],
[
{
...
}
]
]
],
"design": { // additional data for formatting the table in the GUI,
// see API reference for details
"display": {
"customCssClass": "",
"isBordered": true,
"isCondensed": false,
"isHover": false,
"isStriped": false
},
"layout": { // layout within the parent element, default values
"marginBottom": "0px",
"marginLeft": "0px",
"marginRight": "0px",
"marginTop": "0px"
}
}
}
COLUMNS
Columns are not used in the example schema.
Each column may contain a number of other layouts.
To replace the table from the example with a columns layout, you need the following object:
{
"type": "COLUMNS",
"columns": [ // array of columns
[ // first column from the left
{ // first data entry from top
"fieldName": "firstName",
"type": "SINGLE"
}
{ // second data entry
"fieldName": "lastName",
"type": "SINGLE"
}
],
[ // second column
{
...
},
{
...
}
]
],
"design": { // additional data for formatting the columns in the GUI,
// see API reference for details
"display": {
"customCssClass": "",
"columnProperties": [
{
"column": 1,
"width": "50%"
},
{
"column": 2,
"width": "50%"
}
],
"columnGap": ""
},
"layout": {
"marginTop": "0px",
"marginRight": "0px",
"marginBottom": "0px",
"marginLeft": "0px"
}
}
}