Configuring push notifications (React Native)
Configuring Firebase
Google Firebase Cloud Messaging is necessary to handle push notifications sent from Synerise.
- Follow the instructions in this article.
- Integrate the Firebase project with Synerise. See this article.
Setting up - Android
Requirements
After configuring Firebase, add the google-services.json
file to your project/android/app catalog.
Firebase Cloud Messaging integration
-
Add the google-services dependency to your project’s
build.gradle
file.dependencies { ... classpath 'com.google.gms:google-services:4.3.3' ... }
-
Configure the application’s
build.gradle
as follows:dependencies { implementation fileTree(dir: "libs", include: ["*.jar"]) implementation "com.facebook.react:react-native:+" // from node_modules // FCM implementation "com.google.firebase:firebase-messaging:20.0.1" implementation "com.google.android.gms:play-services-base:17.1.0" implementation "com.google.firebase:firebase-core:17.2.1" implementation "com.google.firebase:firebase-analytics:17.2.1" ... }
-
Make sure that at the end of the application gradle file, you add plugin: ‘com.google.gms.google-services’
-
In your MainApplication class, include
setPushListener
to listen for the changes of the Firebase token.@Override public void onCreate() { super.onCreate(); SoLoader.init(this, /* native exopackage */ false); getReactNativeHost().getReactInstanceManager().createReactContextInBackground(); RNNotifications.setPushListener(new OnRegisterPushListener() { @Override public void onRegisterPushRequired() { FirebaseInstanceId.getInstance().getInstanceId().addOnSuccessListener(instanceIdResult -> { String refreshedToken = instanceIdResult.getToken(); Log.d(TAG, "Refreshed token: " + refreshedToken); RNNotifications.setRegistrationToken(refreshedToken); }); } }); }
Receiving push notifications
In order to handle Synerise push notifications, you must pass the incoming push payload to the Synerise SDK.
- Create a class extending
FirebaseMessagingService
:public class MyFirebaseMessagingService extends FirebaseMessagingService { private static final String TAG = MyFirebaseMessagingService.class.getSimpleName(); @Override public void onMessageReceived(@NonNull RemoteMessage remoteMessage) { super.onMessageReceived(remoteMessage); Map<String, String> data = remoteMessage.getData(); RNNotifications.onNotificationReceive(data); } @Override public void onNewToken(String refreshedToken) { super.onNewToken(refreshedToken); Log.d(TAG, "Refreshed token: " + refreshedToken); if (refreshedToken != null) { RNNotifications.setRegistrationToken(refreshedToken); } } }
- To enable banners, handle the intent when the app starts. You can do it in your
MainActivity
by callingRNNotifications.onNotificationReceive
in youronCreate
andonNewIntent
.@Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); RNNotifications.onNotificationReceive(getIntent().getExtras()); } @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); RNNotifications.onNotificationReceive(intent.getExtras()); }
Setting up - iOS
Requirements
Configure handling Push Notifications in your application. See Apple Notifications.
Firebase Cloud Messaging integration
-
Import
RNNotifications.h
import react-native-synerise-sdk
-
Extend the Firebase Messaging Delegate so our SDK can receive the Firebase token that is required to deliver push notifications from Synerise.
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool { FirebaseApp.configure() Messaging.messaging().delegate = self if #available(iOS 10, *) { UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { (granted, error) in } } else { let settings = UIUserNotificationSettings(types: [.alert, .badge, .sound], categories: nil) application.registerUserNotificationSettings(settings) } application.registerForRemoteNotifications() } // MARK: - MessagingDelegate func messaging(_ messaging: Messaging, didReceiveRegistrationToken fcmToken: String) { RNNotifications.didChangeRegistrationToken(fcmToken) }
Important: Make sure that the Firebase token is always up-to-date. When it changes, useRNNotifications.didChangeRegistrationToken(registrationToken:)
again.
Receiving push notifications
The following code shows how to receive push notifications in the AppDelegate.h
and pass these to the React Native part of the application:
// iOS 9
// Push Notifications
// Silent Push Notifications
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable : Any], fetchCompletionHandler completionHandler: @escaping (UIBackgroundFetchResult) -> Void) {
RNNotifications.didReceiveNotification(userInfo)
completionHandler(.noData)
}
func application(_ application: UIApplication, handleActionWithIdentifier identifier: String?, forRemoteNotification userInfo: [AnyHashable : Any], completionHandler: @escaping () -> Void) {
RNNotifications.didReceiveNotification(userInfo, actionIdentifier:identifier)
completionHandler()
}
// iOS 10 and above
// Push Notifications
// MARK: - UNUserNotificationCenterDelegate
@available(iOS 10.0, *)
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
RNNotifications.didReceiveNotification(response.notification.request.content.userInfo, actionIdentifier:response.actionIdentifier)
completionHandler()
}
@available(iOS 10.0, *)
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
RNNotifications.didReceiveNotification(notification.request.content.userInfo)
completionHandler(UNNotificationPresentationOptions.init(rawValue: 0))
}
Extensions for push notifications
Notification Service Extension
Synerise Notification Service Extension is an object that adds the notification functionality to the SDK.
It implements the following operations:
- Decrypting Simple Push communication data (if encryption is enabled).
- Tracking events from Simple Push communication.
- Adding action buttons to Simple Push communication (if the communication contains any).
- Improving the appearance of Simple Push communication (Rich Media - Single Image) with an image thumbnail.
Notification Service Extension should be implemented in the native part of the application. Follow the instructions in this article.
Rich Media Notification Content Extensions
Synerise Rich Media Notification Content Extension is an object that allows rendering your own appearance of a push notification when the notification is expanded (by tapping the notification).
Synerise Rich Media Notification Content Extensions should be implemented in the native part of the application. Follow the instructions in this article.
Set up Firebase FCM token registration for Synerise SDK
Get Firebase FCM token from the native part of the application so our SDK can receive the Firebase token that is required to deliver push notifications from Synerise. Make sure that the Firebase FCM token is always up-to-date by implementing the onRegistrationRequired() method.
Synerise.Notifications.setListener({
onRegistrationToken: function(token) {
Synerise.Notifications.registerForNotifications(token, true, function() {
// success
}, function(error) {
// failure
});
},
onRegistrationRequired: function() {
let registrationToken = getLastPushRegistrationToken();
let mobilePushAgreement = true; // true or false, should depend on device permissions and customer's agreement in the application
Synerise.Notifications.registerForNotifications(registrationToken, mobilePushAgreement, function() {
// success
}, function(error) {
// failure
});
}
//...
});
Configure Notification Encryption
Android
See Configure Notification Encryption.
iOS
See Synerise Notification Service Extension and Configure Notification Encryption.
Application implementation
In the application, you must set encryption
to true
in the SDK initializer or in the SDK settings.
// The first way
// WARNING: This option must be configured before Synerise SDK is initialized!
Synerise.Settings.notifications.encryption = true;
// The second way
Synerise.Initializer()
.withClientApiKey('XXXXXXX-XXXX-XXXX-XXXX-XXXXXXXXXXXX')
.withBaseUrl(null)
.withDebugModeEnabled(true)
.withCrashHandlingEnabled(true)
.withSettings({
notifications: {
enabled: true,
encryption: true
}
//...
})
.init()
Handling incoming push notifications
Synerise payload
The following code shows how to handle push notifications:
Synerise.Notifications.setListener({
//...
onNotification: function(payload, actionIdentifier) {
if (Synerise.Notifications.isSyneriseNotification(payload)) {
Synerise.Notifications.handleNotification(payload, actionIdentifier);
}
}
//...
});
Custom payload
You may send both custom push notifications and custom campaigns in Synerise. The code below of one sample delegate method checks the notification origin and then handles it.
Synerise.Notifications.setListener({
//...
onNotification: function(payload, actionIdentifier) {
if (Synerise.Notifications.isSyneriseNotification(payload)) {
Synerise.Notifications.handleNotification(payload, actionIdentifier);
} else {
// Handle other notification in your own way
}
}
//...
});
Encrypted payloads
If you handle the Synerise notification, you do not have to do anything. The SDK decrypts Synerise notification’s payload:
- In Notification Service Extension for push notifications
- In the SDK, after invoking
Synerise.Notifications.handleNotification(payload, actionIdentifier)
for silent push notifications
Otherwise, if it is a custom encrypted push notification sent by Synerise, or you need decrypt data from the push notification, there are two methods for dealing with it:
Synerise.Notifications.isNotificationEncrypted(payload)
- checks if the notification payload is encrypted by Synerise.Synerise.Notifications.decryptNotification(payload)
- decrypts a notification payload.
Synerise.onReady(function() {
//...
onNotification: function(payload, actionIdentifier) {
if (Synerise.Notifications.isSyneriseNotification(payload)) {
let isNotificationEncrypted = Synerise.Notifications.isNotificationEncrypted(payload)
var decryptedPayload
if (isNotificationEncrypted) {
decryptedPayload = Synerise.Notifications.decryptNotification(payload)
} else {
decryptedPayload = payload
}
Synerise.Notifications.handleNotification(decryptedPayload, actionIdentifier)
}
}
//...
})
})
Synerise.Notifications.decryptNotification(payload)
method returns raw data when the payload is not encrypted. If the operation fails, the method returns null.Handling actions from push notifications
- Read more about types of actions in campaigns
- Read more about handling actions from push notifications
Additional in-app alert from push notifications
The React Native SDK on iOS devices can display an additional alert in the application after a push notification is received. See this article to read more about this feature.
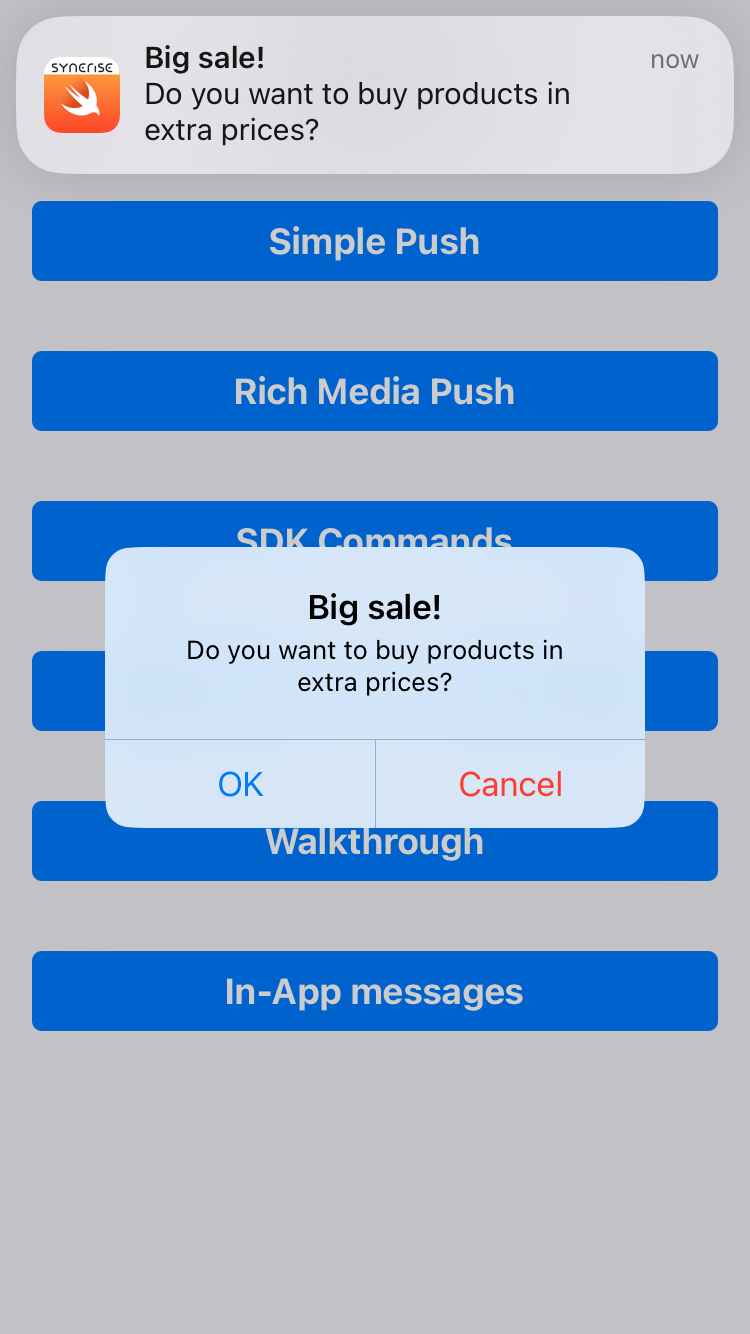
Limitations compared to native platforms
Due to platform limitations, not all notification functionalities may work as in native SDKs.